Steps in getting started
- Download Java
- Download Java SDK
- Get an Integrated Development Environment (IDE)
- Start a project within the IDE
- Code up "Hello World" and run the code
It is categorized by its (string[]arg) as a parameter to the function
public class MyMainFunction {
/* Java main function example */
public static void main(String[] args) {
System.out.println("Hello World");
}
}
In this example, we are printing out "Hello world" to the console when we run the program.
Printing to the console
Functions are actually called methods in Java. Here is an example of how to declare a Java method.
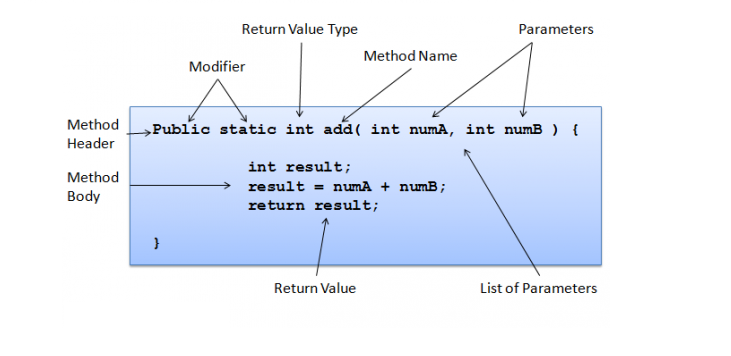
public static void myFunction(String name, int age) {
// function code
}
Java is known as an object oriented programming language.
This means that it is easy to represent entities as objects by using classes and encapsulation.
An example of this might be a student class to represent a student.
public class Student {
/* Student properties */
private String name;
private int age;
/* Constructor */
public Student(String name, int age) {
this.name = name;
this.age = age;
}
/* Getter method */
public String getName() {
return name;
}
/* Setter method */
public void setName(String name) {
this.name = name;
}
}